Python is renowned for its simplicity and readability, making it a popular choice for beginners and experts alike. One of the foundational data structures in Python is the list, a versatile and powerful tool for storing and manipulating data. In this blog, we will explore the fundamentals of lists, how to create and manipulate them, and some advanced techniques to harness their full potential.
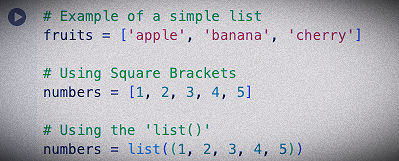
What is a List in Python?
A list in Python is an ordered collection of elements that can hold a variety of data types. Unlike arrays in other programming languages, Python lists are dynamic, meaning their size can change, and they can store elements of different types. Lists are defined using square brackets [] and elements are separated by commas.
# Example of a simple list
fruits = ['apple', 'banana', 'cherry']
In this example, fruits is a list containing three string elements.
Creating Lists in Python
You can create a list in Python using several methods:
# Using Square Brackets
numbers = [1, 2, 3, 4, 5]
# Using the 'list()'
numbers = list((1, 2, 3, 4, 5))
# Empty Lists
empty_list = []
empty_list = list()
Accessing List Elements in Python
Lists are ordered, which means each element has a specific position or index. Python uses zero-based indexing, so the first element is accessed using index 0.
# Accessing elements
print(fruits[0]) # Output: apple
print(fruits[1]) # Output: banana
You can also use negative indexing to access elements from the end of the list.
# Negative indexing
print(fruits[-1]) # Output: cherry
print(fruits[-2]) # Output: banana
Modifying Lists in Python
Lists are mutable, meaning you can change their content without changing their identity.
# Changing Elements
fruits[0] = 'orange'
print(fruits) # Output: ['orange', 'banana', 'cherry']
Adding Elements to Lists in Python
Using append(): Adds an element to the end of the list.
fruits.append('grape')
print(fruits) # Output: ['orange', 'banana', 'cherry', 'grape']
Using insert(): Inserts an element at a specific position.
fruits.insert(1, 'kiwi')
print(fruits) # Output: ['orange', 'kiwi', 'banana', 'cherry', 'grape']
Using extend(): Extends the list by appending elements from another list.
more_fruits = ['mango', 'pineapple']
fruits.extend(more_fruits)
print(fruits) # Output: ['orange', 'kiwi', 'banana', 'cherry', 'grape', 'mango', 'pineapple']
Removing Elements from Lists in Python
Using remove(): Removes the first occurrence of a value.
fruits.remove('banana')
print(fruits) # Output: ['orange', 'kiwi', 'cherry', 'grape', 'mango', 'pineapple']
Using pop(): Removes the element at the specified position and returns it. If no index is specified, it removes the last item.
removed_fruit = fruits.pop(2)
print(removed_fruit) # Output: cherry
print(fruits) # Output: ['orange', 'kiwi', 'grape', 'mango', 'pineapple']
Using del: Deletes elements by index or slices.
del fruits[1]
print(fruits) # Output: ['orange', 'grape', 'mango', 'pineapple']
Using clear(): Removes all elements from the list.
fruits.clear()
print(fruits) # Output: []
List Comprehensions in Python
List comprehensions provide a concise way to create lists. They can include conditions and transform data in a readable and efficient manner.
# Creating a list of squares
squares = [x**2 for x in range(10)]
print(squares) # Output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]
# Filtering with list comprehensions
even_squares = [x**2 for x in range(10) if x % 2 == 0]
print(even_squares) # Output: [0, 4, 16, 36, 64]
Common List Methods in Python
Here are some useful methods that can be used with lists:
len(list): Returns the number of elements in the list.
list.count(value): Returns the number of occurrences of a value.
list.sort(): Sorts the list in ascending order (modifies the list in place).
list.reverse(): Reverses the list in place.
list.index(value): Returns the index of the first occurrence of a value.
In conclusion, lists in Python are a versatile and essential tool, allowing developers to store and manipulate data efficiently. Whether you're just starting with Python or looking to refine your skills, understanding and utilizing lists is fundamental to your programming journey. As you continue to explore Python, you'll find lists to be invaluable in a wide range of applications, from simple data storage to complex data processing tasks.
Keep experimenting with different list operations and techniques, and you'll soon master this powerful data structure. Happy coding!
Comments