In this blog post, we'll explore what the Fibonacci series is and how to generate it using Python. We'll look at a few different methods, including a simple iterative approach, a recursive approach, and a more efficient approach using memoization.
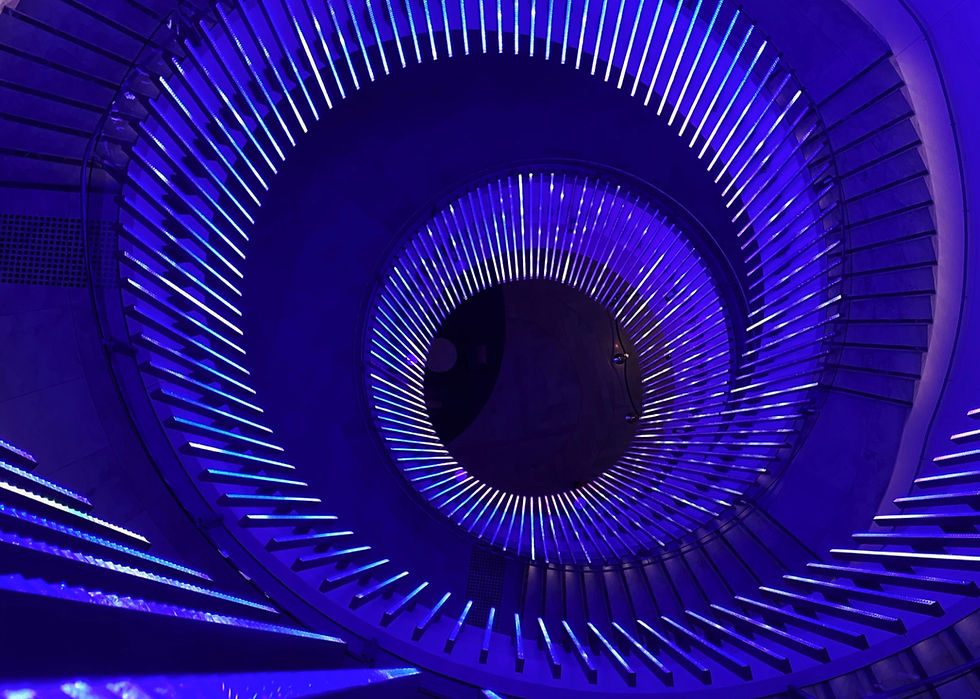
What is the Fibonacci Series?
The Fibonacci series is a sequence of numbers in which each number is the sum of the two preceding ones, typically starting with 0 and 1. This series appears in various areas of mathematics and science, making it an interesting and valuable concept to understand and implement in programming. The Fibonacci series starts with the numbers 0 and 1. From there, each subsequent number is the sum of the previous two. Here are the first few numbers in the Fibonacci series:
0, 1, 1, 2, 3, 5, 8, 13, 21, 34, ...
The mathematical formula for the Fibonacci sequence is:
F(n) = F(n-1) + F(n-2)
Where:
F(0) = 0
F(1) = 1
Importance of Fibonacci Series
The Fibonacci series holds significant importance across various domains of mathematics, science, and nature. Its ubiquitous presence in natural phenomena, such as the arrangement of leaves on a stem, the branching of trees, and the flowering of artichokes, highlights its intrinsic connection to the natural world. In mathematics, the Fibonacci sequence provides insights into number theory and has applications in algorithms and computational processes, particularly in recursive algorithms and dynamic programming. Moreover, the series is pivotal in financial markets, where Fibonacci retracement levels are used in technical analysis to predict potential price movements. The beauty and simplicity of the Fibonacci series, coupled with its profound applicability in diverse fields, make it a fascinating and essential concept to study and understand.
Generating the Fibonacci Series in Python; Iterative Approach
The iterative approach is straightforward and efficient. We use a loop to generate the series.
def fibonacci_iterative(n):
fib_series = [0, 1]
while len(fib_series) < n:
next_value = fib_series[-1] + fib_series[-2]
fib_series.append(next_value)
return fib_series[:n]
# Example usage
n = 10
print(f"First {n} Fibonacci numbers: {fibonacci_iterative(n)}")
Output of the above code :
First 10 Fibonacci numbers: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
Generating the Fibonacci Series in Python; Recursive Approach
The recursive approach is more elegant but less efficient for large values of n due to redundant calculations.
def fibonacci_recursive(n):
if n <= 0:
return 0
elif n == 1:
return 1
else:
return fibonacci_recursive(n-1) + fibonacci_recursive(n-2)
# Example usage
n = 10
fib_series = [fibonacci_recursive(i) for i in range(n)]
print(f"First {n} Fibonacci numbers: {fib_series}")
Output of the above code :
First 10 Fibonacci numbers: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
Generating the Fibonacci Series in Python; Using Memoization
To optimize the recursive approach, we can use memoization to store previously calculated values.
def fibonacci_recursive(n):
if n <= 0:
return 0
elif n == 1:
return 1
else:
return fibonacci_recursive(n-1) + fibonacci_recursive(n-2)
# Example usage
n = 10
fib_series = [fibonacci_recursive(i) for i in range(n)]
print(f"First {n} Fibonacci numbers: {fib_series}")
Output of the above code :
First 10 Fibonacci numbers: [0, 1, 1, 2, 3, 5, 8, 13, 21, 34]
Visualizing the Fibonacci Series
Let's visualize the Fibonacci series using a simple plot. We'll use the matplotlib library for this purpose.
import matplotlib.pyplot as plt
# Generate Fibonacci series
n = 10
fib_series = fibonacci_iterative(n)
# Plot the series
plt.plot(fib_series, marker='o')
plt.title(f'First {n} Fibonacci Numbers')
plt.xlabel('Index')
plt.ylabel('Fibonacci Number')
plt.grid(True)
plt.show()
Output of the above code :
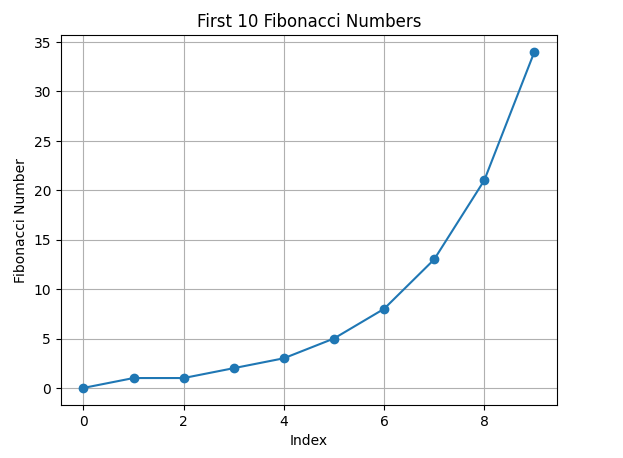
In conclusion, the Fibonacci series is a fundamental concept in mathematics with various applications. In this blog post, we've explored three different methods to generate the Fibonacci series in Python: iterative, recursive, and memoization. Each method has its advantages and trade-offs in terms of simplicity and efficiency.
Understanding and implementing the Fibonacci series is an excellent exercise for improving your problem-solving and programming skills. Try experimenting with different approaches and see how they perform with larger values of n.
Comments