Maps in JavaScript are a collection of key-value pairs where keys can be of any data type. Unlike objects, which only support string or symbol keys, Maps provide more flexibility and have built-in methods for manipulation. In this tutorial, we will explore Maps, their methods, and how to use them effectively in JavaScript.
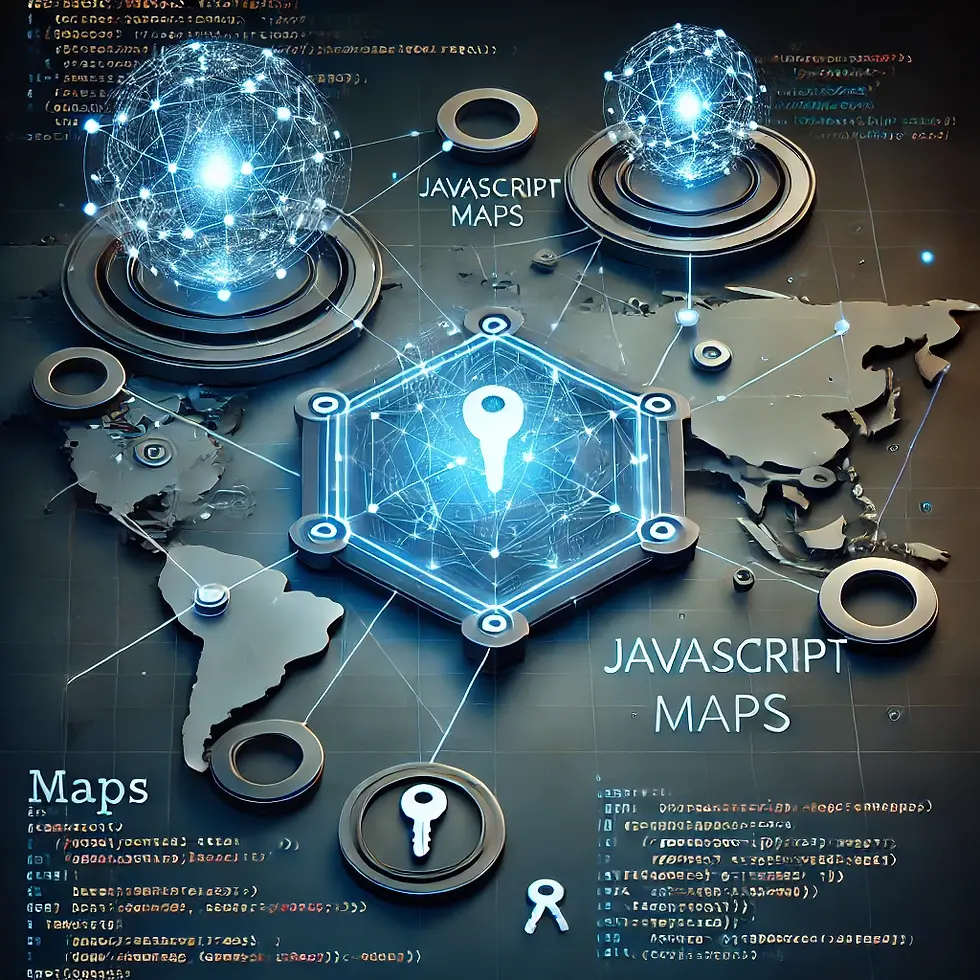
What is a Map in JavaScript?
A Map in JavaScript is an ordered collection of key-value pairs. It maintains the order of entries as they were added, and allows keys of any data type, including objects, numbers, and even other Maps. Maps are particularly useful when you need to store and retrieve data efficiently while maintaining the integrity of key-value relationships. They provide built-in methods for easy manipulation and iteration, making them an excellent alternative to traditional JavaScript objects in certain use cases. Map in JavaScript is an ordered collection of key-value pairs. It maintains the order of entries as they were added, and allows keys of any data type, including objects, numbers, and even other Maps.
Creating a Map
To create a Map, we use the new Map() constructor:
let myMap = new Map();
We can also initialize a Map with values:
let userRoles = new Map([
['Alice', 'Admin'],
['Bob', 'Editor'],
['Charlie', 'Viewer']
]);
Map Methods
Maps in JavaScript come with a variety of built-in methods that allow developers to manipulate and retrieve key-value pairs efficiently. These methods help in managing data dynamically and ensure smooth operations while working with collections. Below are some of the most commonly used Map methods.
1. set(key, value) – Adds or updates an entry
myMap.set('name', 'John');
myMap.set(1, 'one');
console.log(myMap);
2. get(key) – Retrieves the value for a key
console.log(myMap.get('name')); // Output: John
3. has(key) – Checks if a key exists
console.log(myMap.has('age')); // Output: false
4. delete(key) – Removes an entry
myMap.delete('name');
console.log(myMap.has('name')); // Output: false
5. size – Returns the number of elements in the Map
console.log(myMap.size);
6. clear() – Removes all elements
myMap.clear();
console.log(myMap.size); // Output: 0
Iterating Over a Map
Maps provide several ways to iterate over their key-value pairs, making it easy to process and manipulate data. Whether you need to loop through keys, values, or complete entries, JavaScript offers built-in methods to achieve this efficiently.
We can iterate over a Map using different methods:
Using forEach()
userRoles.forEach((value, key) => {
console.log(`${key} - ${value}`);
});
Using for...of
for (let [key, value] of userRoles) {
console.log(`${key} - ${value}`);
}
Getting Keys, Values, and Entries
console.log([...userRoles.keys()]); // Output: ['Alice', 'Bob', 'Charlie']
console.log([...userRoles.values()]); // Output: ['Admin', 'Editor', 'Viewer']
console.log([...userRoles.entries()]);
Map vs Object
When working with key-value pairs in JavaScript, both Maps and Objects offer ways to store and manage data. However, they have fundamental differences in terms of key types, ordering, and performance. Understanding these distinctions will help in choosing the appropriate data structure for a given task.
Feature | Map | Object |
Key Type | Any type | Strings & Symbols |
Order | Maintains insertion order | No guaranteed order |
Iteration | Easier with built-in methods | Requires manual iteration |
Performance | Optimized for frequent additions/removals | Better for fixed structures |
Use Cases of Maps
Maps are widely used in JavaScript applications due to their efficiency and flexibility in handling key-value pairs. They provide a structured way to manage data with unique keys and are particularly useful in scenarios where quick lookups and ordered key-value storage are required.
Storing user roles and permissions.
Caching computed results.
Associating metadata with DOM elements.
Managing configuration settings dynamically.
Conclusion
Maps in JavaScript provide an efficient way to store key-value pairs with better performance and flexibility compared to objects. They offer advantages such as maintaining insertion order, supporting any data type as keys, and having built-in methods for easy data manipulation.
By using methods like set(), get(), and iteration tools, we can manage data effectively in our applications. Maps are particularly useful in cases where frequent additions and removals occur, or when complex key types are required.
Try using Maps in your next JavaScript project and experience the difference! in JavaScript provide an efficient way to store key-value pairs with better performance and flexibility compared to objects. By using methods like set(), get(), and iteration tools, we can manage data effectively in our applications.
Try using Maps in your next JavaScript project and experience the difference!
Comments